Saving reconstruction(s) under a different name#
If the existing outputs options from nabu-slice/nabu-volume are too limited or if you want to copy volume under another name (like to take into account if a filter is used from nabu reconstruction parameters) then you can add after the nabu-slice reconstruction or the nabu-volume reconstruction a python widget to modify the output name or copy the reconstructions. Here is a small examples. We consider a python-script widget added just after the nabu-slice reconstruction widget like:
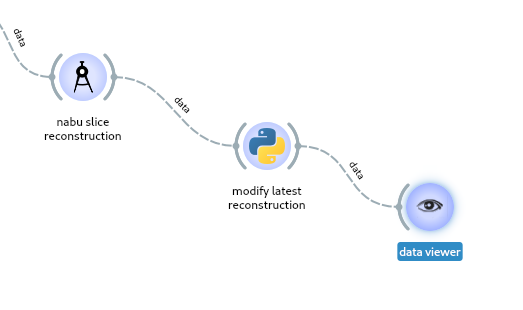
import os
from tomwer.core.volume.volumefactory import VolumeFactory
from tomwer.core.volume.hdf5volume import HDF5Volume
scan = in_data
new_reconstructions = []
# go through all the latest 'nabu slice reconstruction' output
# note: to go through all reconstructed volume (after nabu-volume widget)
# you can use scan.latest_vol_reconstructions instead. Remaining is the same
for identifier in scan.latest_reconstructions:
try:
# retrieve the slice or the volume (same API)
in_volume = VolumeFactory.create_tomo_object_from_identifier(identifier)
in_volume.load()
except Exeption:
print(f"Fail to load {in_volume}. Did the reconstruction failed ?")
else:
# if you want to can retrieve nabu reconstruction parameter used.
nabu_config = scan.nabu_recons_params
# copy the slice to another name (according to nabu parameters)
# in this case we want to know which fbp filter has beem used
filter_name = nabu_config["reconstruction"]["fbp_filter_type"]
# then we create the new file name (in this case we work with hdf5)
output_volume_dir = os.path.dirname(in_volume.file_path)
file_name = os.path.splitext( # split file name and extension
os.path.basename(in_volume.file_path) # retrieve file name (without the path)
)[0]
new_file_path = os.path.join(
output_volume_dir,
f"{scan.scan_dir_name()}_{filter_name()}.hdf5",
)
out_volume = HDF5Volume(
file_path=new_file_path,
data_path=in_volume.data_path,
data=in_volume.data,
metadata=in_volume.metadata,
)
print("new output volume file path is", new_file_path)
out_volume.save()
# register the new reconstruction (ensure downstream processing to work from the new files)
if len(new_reconstructions) > 0:
scan.set_latest_reconstructions(
new_reconstructions
)
out_data = scan
Note
on this example we go through all reconstructed slice using scan.latest_reconstructions. You can do the same for volume. In this case:
the python script widget must be located after a ‘nabu-volume’ widget
you must replace iteration over slice (scan.latest_reconstructions) by iteration over volumes (scan.latest_vol_reconstructions)
set latest reconstructed volume by calling set_latest_vol_reconstructions instead of setting latest slice (scan.set_latest_reconstructions)
Hint
If you are working wth other volume type (TIFFVolume…) please have a look at the tomoscan volume API and the tomoscan volume tutorials Tomwer is using the same API. Just make sure you import the volume class from tomwer.core.volume instead of tomoscan.esrf.volume